In this program we will model some people at a university
using object-oriented techniques. As with Lab 8, the emphasis in this
program is not on transforming input into output, but rather on correctly
implementing a specification.
Let's be upfront about something: many of the requirements of this
program are rather arbitrary. A well-done model of people at a university
would require thousands of lines of code. This is just a brief jaunt into
the world of OOP.
Your driver program should be in a class called University. You should
test the methods of your other classes in the driver. For example, you
could instantiate a Person, output the person using toString(), and have the
person speak (more on each of those below).
The classes you will implement are: Person, Student, GraduateStudent,
and Faculty. You will also use the Grader interface. Grader and a partial
implementation of Person are provided for you.
The class hierarchy for this program is:
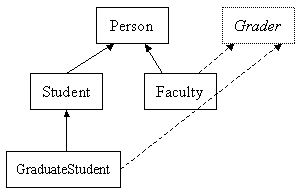
In the above diagram, solid lines indicate subclassing
(the is-a relationship) and dotted lines indicate interface
implementation (the realizes relationship).
Full specifications for Grader and Person are provided
below.
Grader |
int grade(String assignment)
|
Grade an assignment with the given name;
print a message to System.out including the assignment name
and grade given; return the grade. Grade should be between 0
and 100. |
int grade(String assignment,
int maxPoints)
|
Same as above, but grade should be between 0
and maxPoints. |
int regrade(String assignment,
int prevGrade)
|
Regrade an assignment with the given name and
previous grade; print a message to System.out including the
assignment name, previous grade, and new grade; return the
new grade. |
|
Person
|
String name
|
The person's name; default value
= "Unknown" |
int age
|
The person's age; default value =
30 |
|
Person()
|
Default constructor |
Person(String n)
|
Construct a Person with the given
name and the default age |
Person(String n, int a)
|
Construct a Person with the given
name and age |
void speak()
|
Print a message to System.out:
"What's for dinner?" |
String toString()
|
Return all information about the
person as a String |
|
The remaining classes are described below.
- Student
- A student is a person.
- The default age for a student is 20.
- A student has a gpa, which has a default value of 0.0.
- Student should have three constructors: a default, one that
accepts just the student's name, and a third that accepts the
student's name, age, and gpa.
- Students should respond to the speak message as a person would.
- GraduateStudent
- A graduate student is a student
- The default age for a graduate student is 25.
- Graduate students are members of a department, which has a
default value of "Unknown".
- GraduateStudent should have three constructors: a default, one
that accepts just the student's name, and a third that accepts the
student's name, age, gpa, and department.
- Graduate students should speak a message of your choice that is
different from what students and persons speak.
- When graduate students grade an assignment, they assign a random
grade between 70% and 100% of the maximum score possible.
- When graduate students regrade an assignment, they randomly add
between 1 and 10 points to the previous grade, regardless of the
maximum score.
- Faculty
- Faculty are people.
- The default age for faculty is 35.
- Faculty are members of a department, which has a default value
of "Unknown".
- Faculty are either tenured or untenured, and untenured is the
default.
- Faculty should have three constructors: a default, one that
accepts just the faculty's name, and a third that accepts the
faculty's name, age, department, and tenure status.
- Faculty should speak a message of your choice that is different
from what graduate students, students, and persons speak.
- When faculty grade an assignment, they assign a random grade
between 0% and 70% of the maximum score possible.
- When faculty regrade an assignment, they randomly subtract
between 1 and 10 points from the previous grade. However,
they will not assign a grade of less than 0.
Note the following:
- It is unusual to perform input or output in classes like we are writing.
We allow it to make the lab
simpler.
- The toString method should print out all information about an
object. An example: for Faculty, it should print the name, age,
department, and tenure status.
- The Math.random() method is an easy way to generate a random number
in the range [0.0, 1.0). To
generate a random integer in the range [min, max] --
and you may assume that all ranges in this assignment are of this form
-- use this formula:
r = (int) (Math.random() * (max - min + 1) + min);
Hint: this would be a good candidate for a static method in the
University class.
- Another way of generating random numbers is using the nextInt(int n)
method in the Random class.
r = generator.nextInt(max - min + 1) + min;
|